vue3_ref的用法解析
ref是干什么的?
我们知道, vue2是这样配置响应式的:
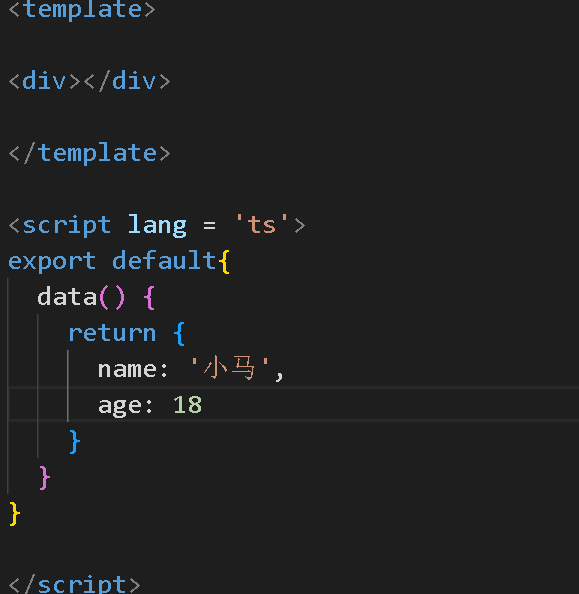
而在vue3中, 我们可以使用ref来配置响应式:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <template>
<div> {{someone}} <button @click="change">按钮</button> </div>
</template>
<script setup lang = 'ts'> import { ref, reactive} from 'vue' const someone =ref( {name : '小马', age : 18}) const change = () => { someone.value.name = '小杨' console.log(someone) } </script> <style scoped>
</style>
|
这里有几个关键的步骤,
1
| import { ref, reactive} from 'vue'
|
我们在修改值的时候: 需要加上.value, 这一步容易被忽略:
1
| someone.value.name = '小杨'
|
官方文档是这样说的:
ref()
takes the argument and returns it wrapped within a ref object with a .value
property:
翻译一下:
Ref ()获取参数并将其包装在具有. value 属性的 ref 对象中返回,如果不适用.value的话, 会报错:
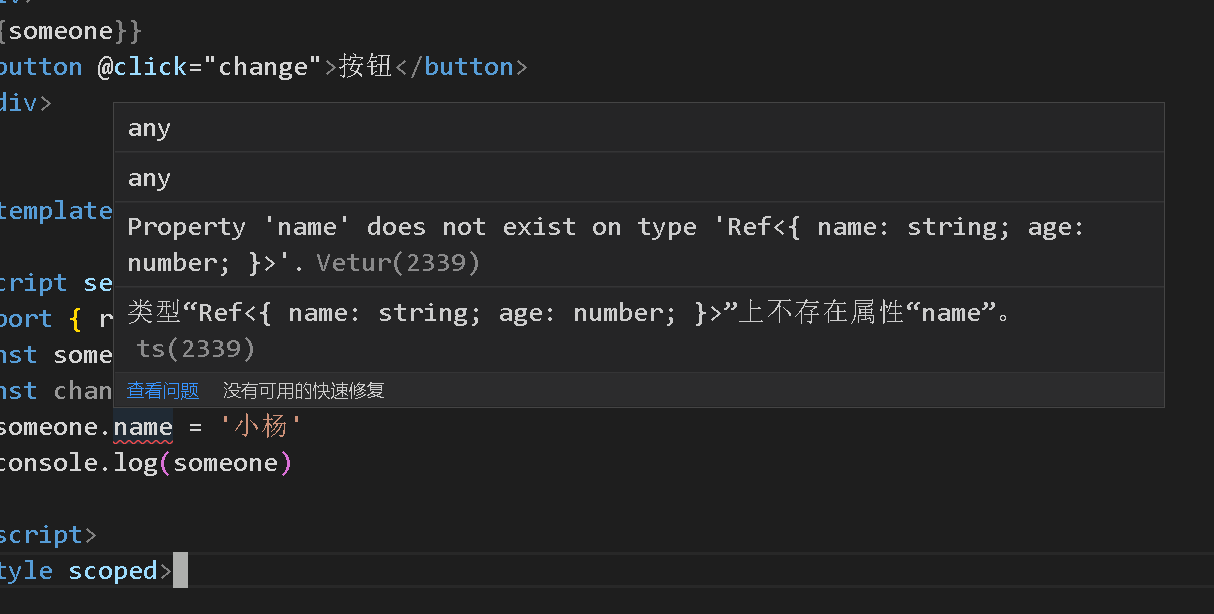
isRef
isRef用于判断一个对象是不是Ref对象, 它的返回值是一个布尔值
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <template>
<div> {{someone}} <button @click="change">按钮</button> </div>
</template>
<script setup lang = 'ts'> import { ref,isRef, reactive} from 'vue' const someone = ref({name: '小桃', age : 20}) const otherone = {name : '大肠', age: 21} const change = () => { console.log(isRef(someone)) console.log(isRef(otherone)); } </script> <style scoped>
</style>
|
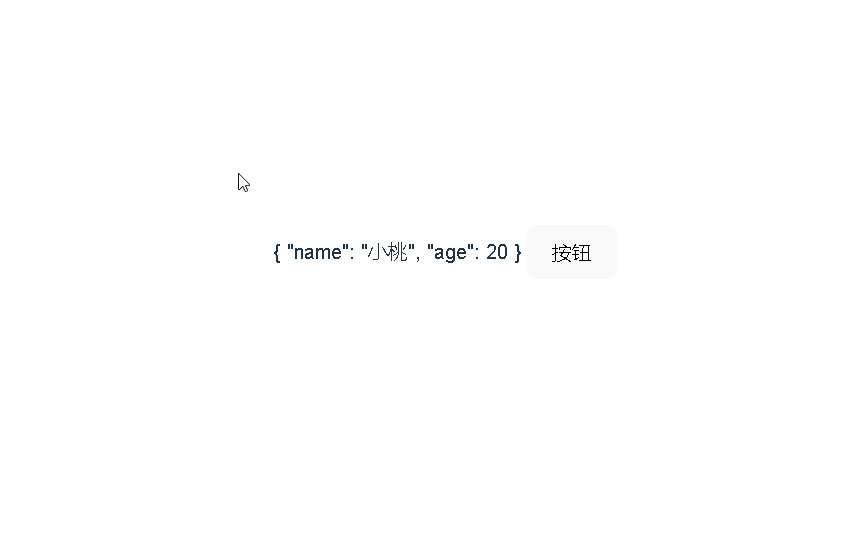
当我点击按钮之后,控制台返回下面的结果:
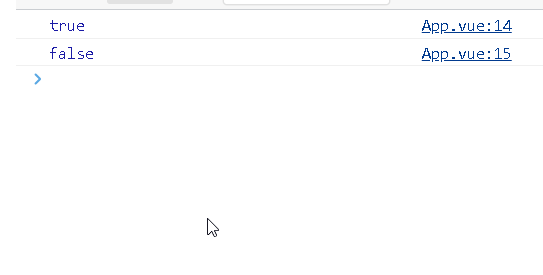
shallowRef
这个是做浅层响应式的, ref是做深层次的:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| <template>
<div> ref:{{someone}} <br> shallowRef: {{otherone}} <br> <button @click="change">按钮</button> </div>
</template>
<script setup lang = 'ts'> import { ref,shallowRef, reactive} from 'vue' const someone = ref({name: '小桃', age : 20}) const otherone = shallowRef({name : '大肠', age: 21}) const change = () => { otherone.value.name = '大肠2' console.log(otherone)
} </script> <style scoped>
</style>
|
执行上面的代码:
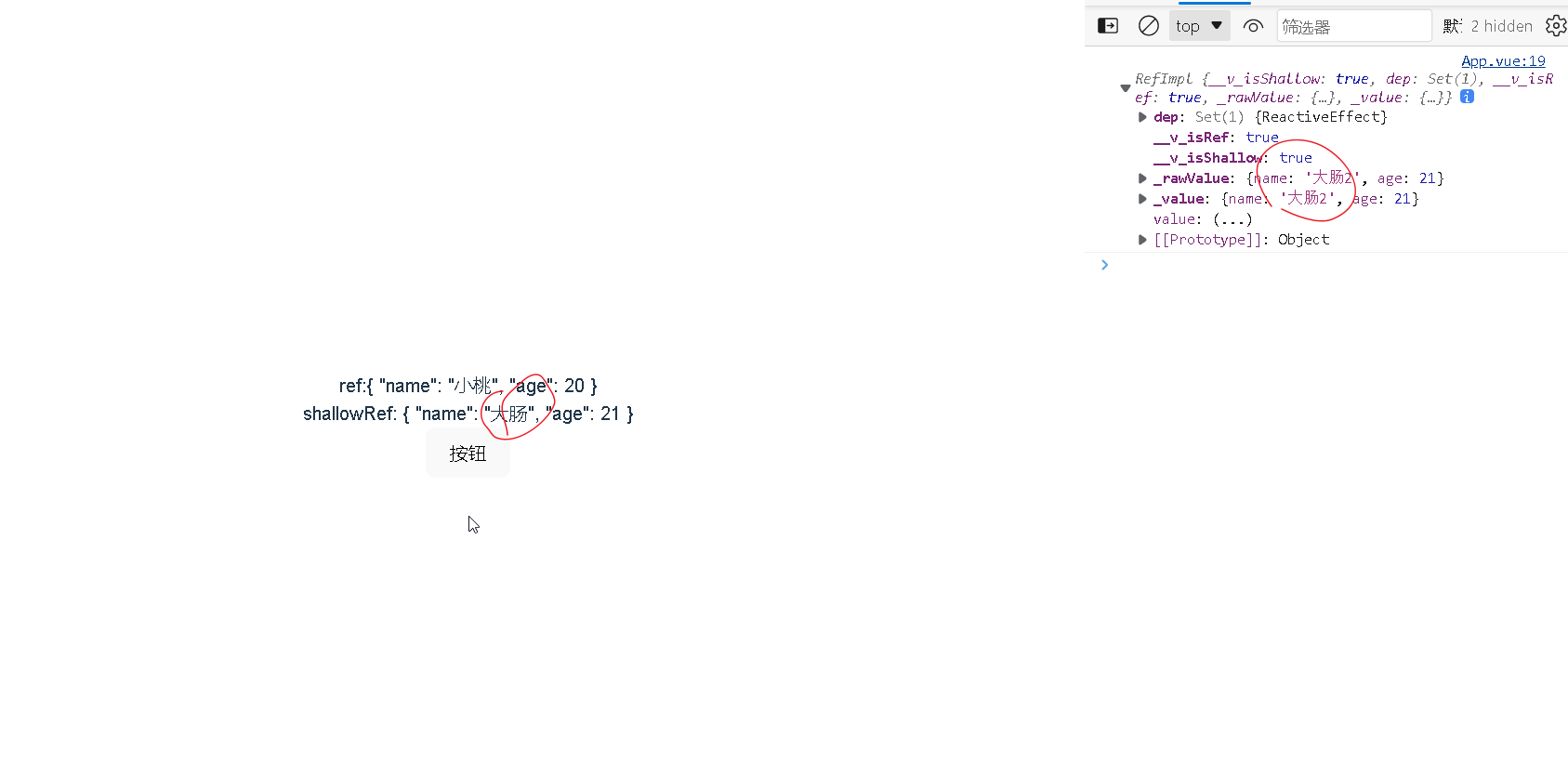
在页面中没有发生变化, 但是在控制台中发生了变化, 这个就是shallowRef的作用, 做浅层响应, 但是如果你在change函数中这个写:
1 2 3 4 5 6 7
| const change = () => { otherone.value = { name : '大肠2' } console.log(otherone)
}
|
这个时候,视图就会更新了, 因为你可以使用value去让它响应
注意:ref和shallowRef是不能一块去写的, 它们会相互影响
triggerRef
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| <template>
<div> ref:{{someone}} <br> shallowRef: {{otherone}} <br> <button @click="change">按钮</button> </div>
</template>
<script setup lang = 'ts'> import { ref,shallowRef, reactive,triggerRef} from 'vue' const someone = ref({name: '小桃', age : 20}) const otherone = shallowRef({name : '大肠', age: 21}) const change = () => { otherone.value.name = '大肠2' triggerRef(otherone)
} </script> <style scoped>
</style>
|
以上方法,也可以通过triggerRef来实现 ,triggerRef会强制更新依赖
ref获取dom元素:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| <template>
<div ref = 'dom'> 我是一个dom元素 </div> <button @click="change">按钮</button> </template>
<script setup lang = 'ts'> import { ref,shallowRef, reactive,triggerRef} from 'vue' const dom = ref<HTMLDivElement>() const change = () => { console.log(dom.value?.innerText);
} </script> <style scoped>
</style>
|
运行结果:
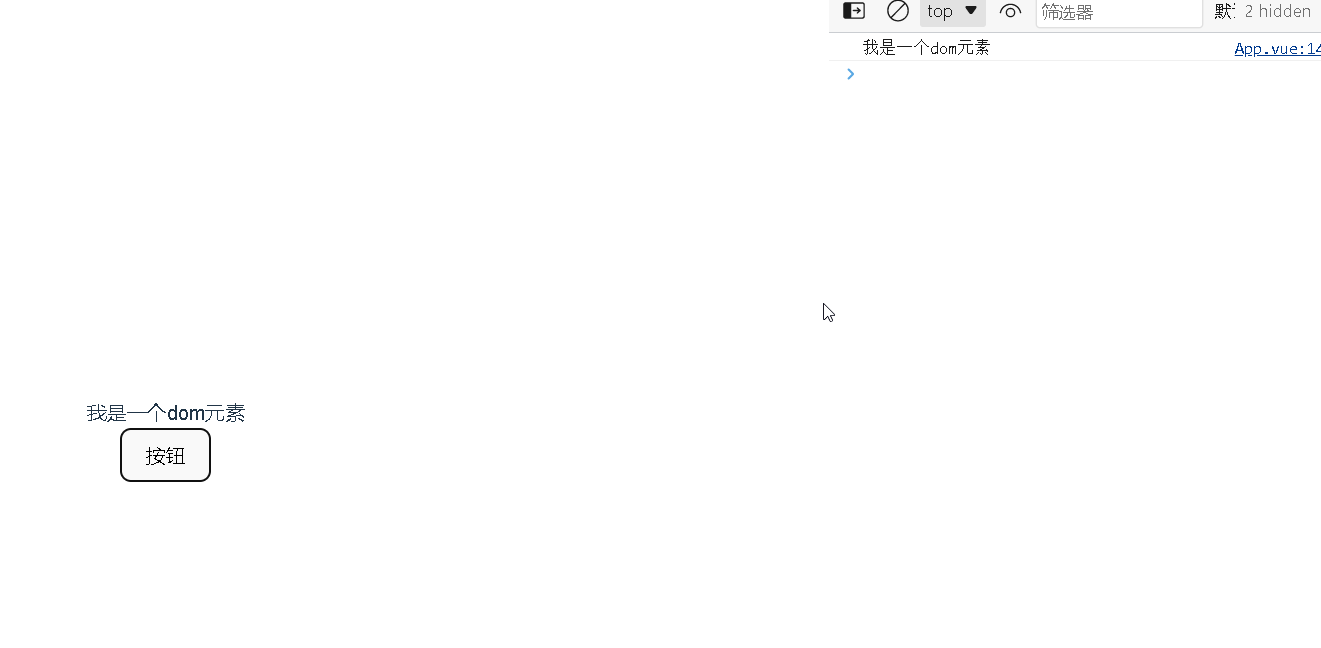